Joseph Vissarionovich Dzhugashvili changed his name to Stalin at a very young age. Stalin derives from Steel, emphasizing the strength of character. And it seems like a young Georgia-born revolutionary perfectly knew that Stalin would be the best fit for the role that he was going to play in history. Stalin belongs to the same generation of politics as Winston Churchill, Adolf Hitler, and Franklin D. Roosevelt. His role in history is full of contradictions. While leading USSR to the victory in WW2, Stalin ruled as dictator causing repressions and a considerable amount of deaths inside the country.
Joseph Dzhugashvili was born on December 18, 1879, in the city of Gori, Georgia. This territory was a part of the Russian Empire then. Joseph’s parents were shoemaker Vissarion Dzhugashvili, and a laundress Ketevan Geladze.
There’s a theory that links childhood traumas and illness with character development. Young Joseph had plenty of them. At the age of 7, he contracted smallpox, that covered his face with pockmarks. And now an interesting one: Stalin’s face looks smooth on all of these historical pictures. It is a result of the retouching. Stalin cared about his images and ordered to hide the pockmark scars on his face.
He also had congenital disabilities: fused second and third toes on his left foot. In 1885, Joseph suffered a phaeton accident. He severely injured his arm and leg; he couldn’t fully bend his left arm after that accident, and it seemed shorter than his right one.
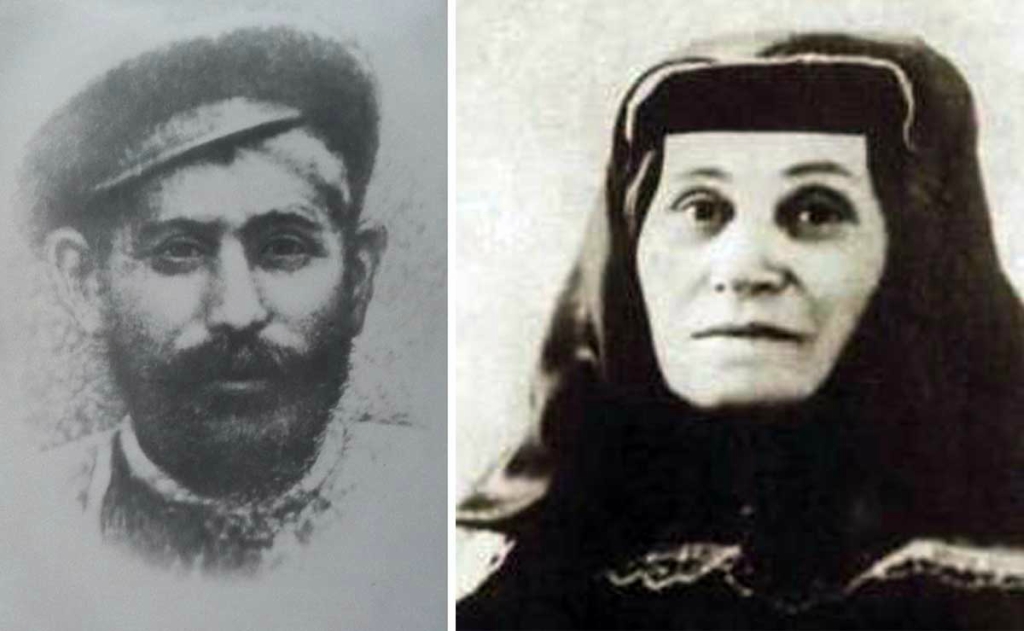
Vissarion and Ketevan Djugashvilli
The drunken story of Joseph’s father
Stalin’s father Vissarion (Beso) was a shoemaker of the village of Didi-Lilo, Tiflis (modern Tbilisi) province. He was always drunk and was reported to local police for the brutal assaults of his wife Ketevan and little Coco (a nickname of young Stalin). There is a story that Joseph tried to protect his mother from his drunk father. A kid threw a knife at Vissarion and ran away. According to the memories of the son of a policeman in Gori, on another occasion, Vissarion broke into the house where Ketevan and little Coco were covering. He beat up both of them, damaging the head of his son.
Joseph was the third son of the family. His elder brothers died at a very young age. The shoemaking business of Stalin’s father didn’t do well. The family frequently relocated, seeking a better job. Ultimately, Vissarion left his wife and tried to take his Joseph with him. Ketevan didn’t let him do so. Joseph was eleven years old when his father “died in a drunken fight.” Someone stabbed him with a knife. No one could keep young kid from spending time in the streets of Gori in a lousy company of local thugs and outlaws.
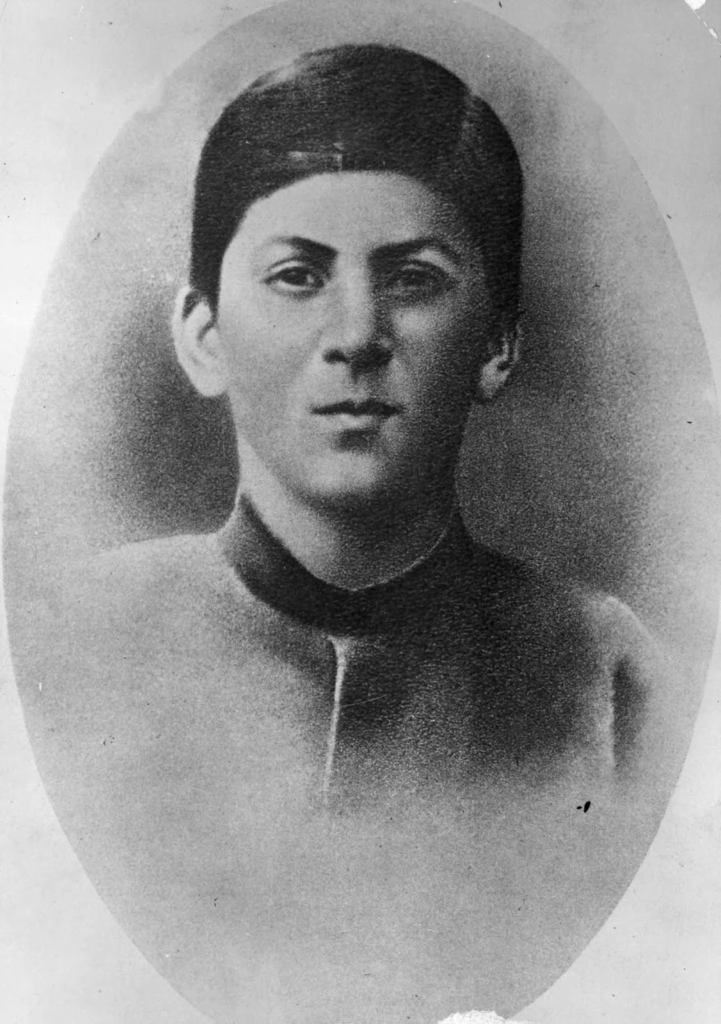
Young Stalin at the age of 15.
Motherlove almost saved Joseph.
Ketevan Georgievna, a mother of Stalin, knew the dark side of his son. She saw how the father’s cruelty corrupted the kid’s character, making his character hideous and evil. Ketevan was a hard-working woman who often punished her only child but loved him at the same time. Stalin’s childhood friend David Machavariani said that “Kato surrounded Joseph with excessive maternal love and, like a mother-wolf, protected him from everyone and everything. She exhausted herself with work to make her darling child happy.”
Historians claim that Ketevan knew about the evil side of Joseph’s character and tried to compensate it with religious education. She even hoped that her son would become a priest. Ketevan tried to assign Joseph to study at the Gori Orthodox Theological School. However, Stalin didn’t speak Russian at all and failed exams. Priest Christopher Charkviani taught Joseph the Russian language during several next years. Stalin spent one year in the Orthodox school and, by the end of the first year, decided he did not believe in God.
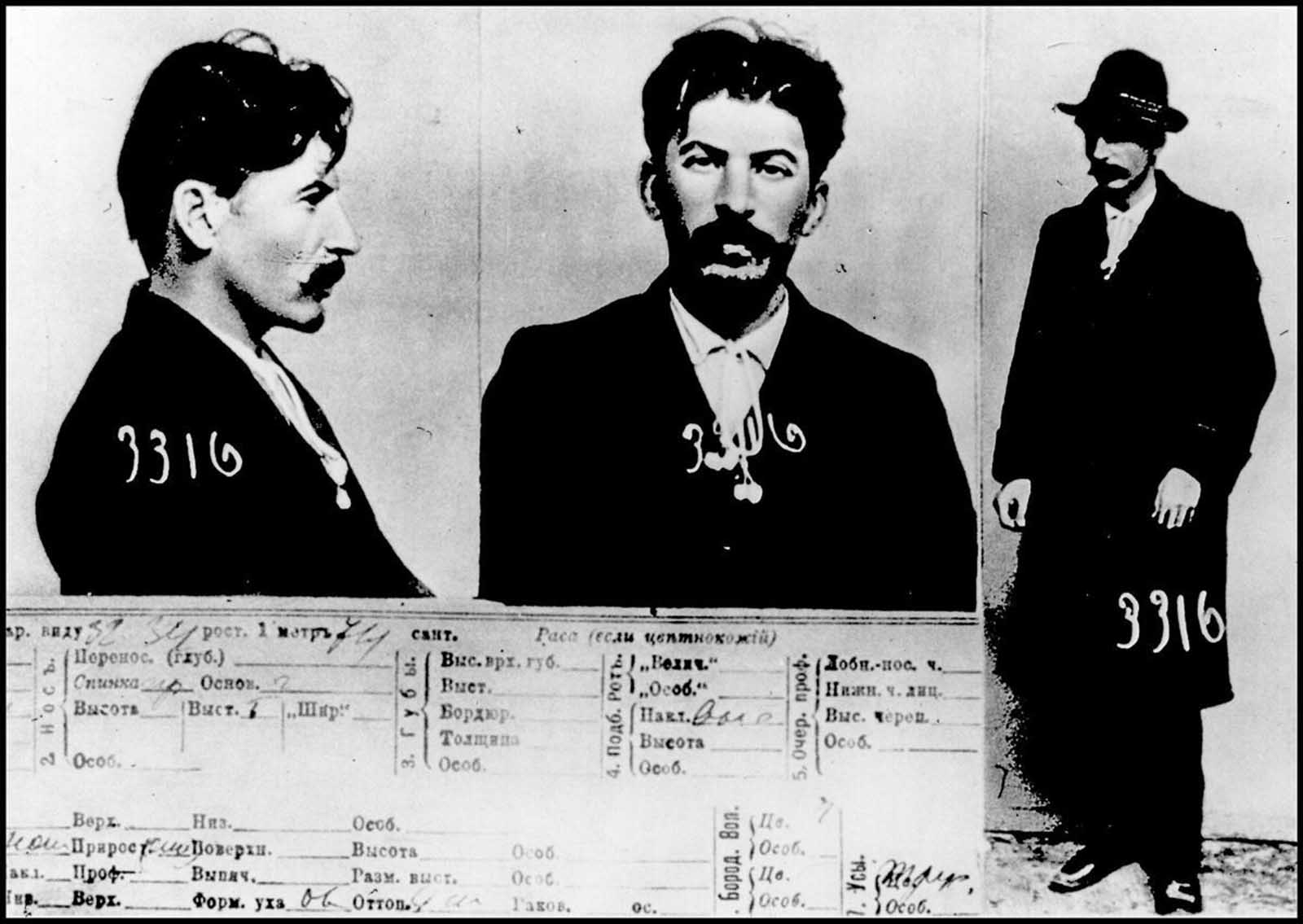
The mugshot and the police card on “I. V. Stalin.” Archives of the police in Saint Petersburg, 1911
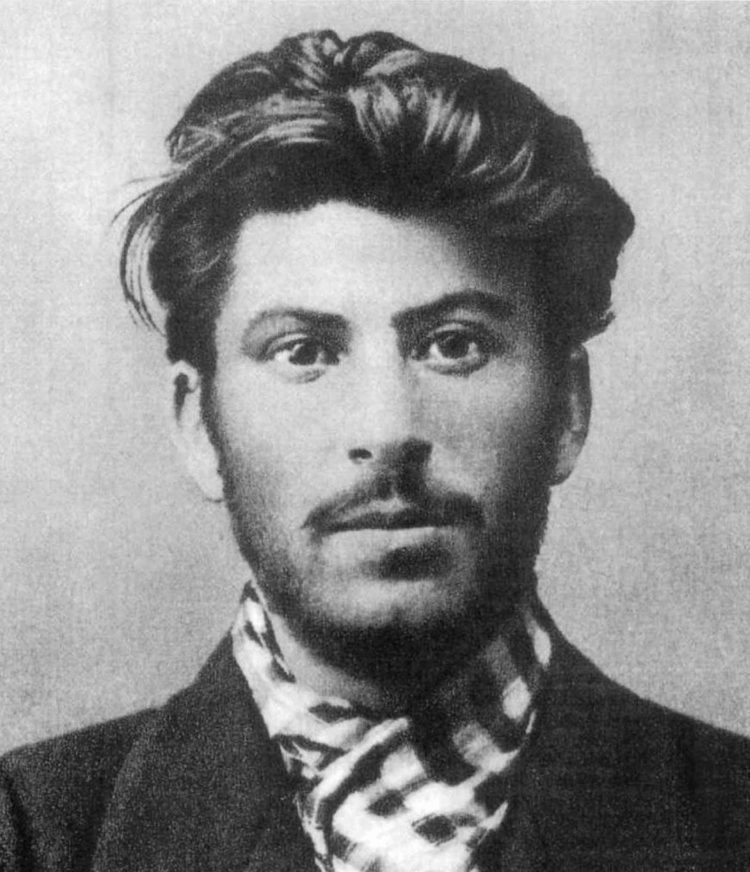
Joseph Stalin at the age of 23
Young Stalin, a gifted student of Marx
Joseph passed the entrance exams and was enrolled in the Orthodox Tiflis Theological Seminary in September 1894. It was a place where Stalin contacted the Marxist Communistic Ideology for the first time. Stalin recalled: “I entered the revolutionary movement at the age of 15 when I got in touch with the underground groups of Russian Marxists who were then living in Transcaucasia. These groups had a great influence on me and instilled in me a taste for underground Marxist literature.”
According to the English historian Simon Sebag-Montefiore, Joseph was an extremely gifted student. Stalin received excellent grades in all subjects: mathematics, theology, Greek, and Russian. Stalin liked poetry and even wrote a few poems in Georgian.
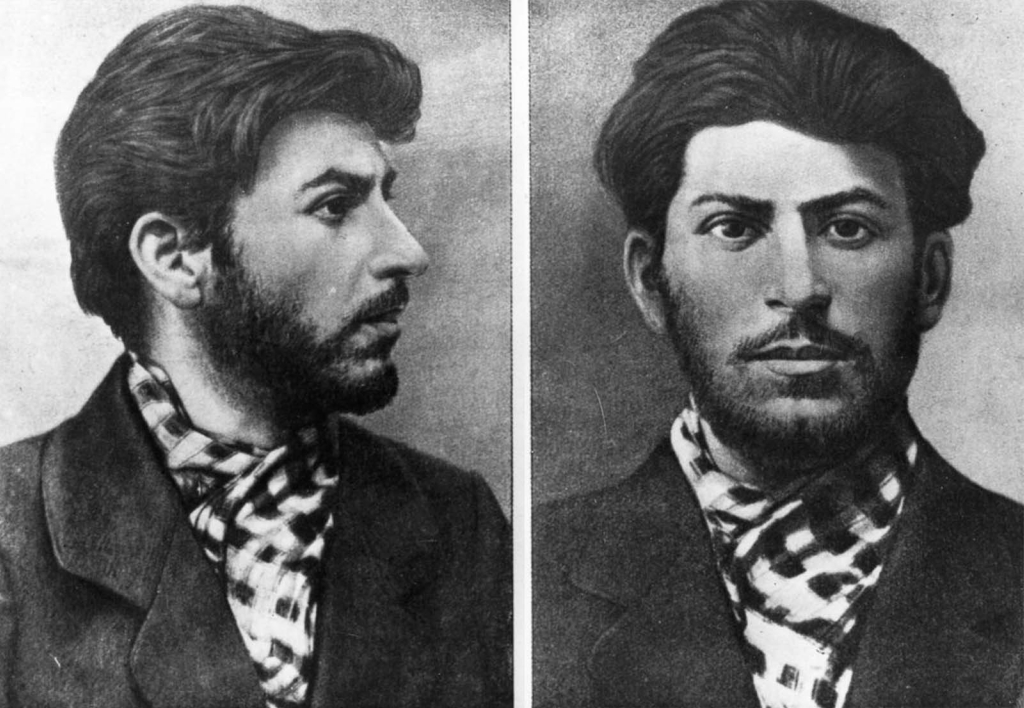
The first mugshot from the police archive, 1908
Stalin had gained the experience of a propagandist in 1898, during a meeting with workers at the apartment of the revolutionary Vano Sturua. He organized an association of the young railroad workers and began to teach them Marxist ideas. In August, Joseph joined the Georgian Social-Democratic organization “Mesame-dasi”. Young Stalin formed the core of the revolutionary minority of this organization, the majority of which supported the positions of “legal Marxism” and inclined towards nationalism.
On May 29, 1899, in his fifth year of study, Stalin was expelled from the seminary “for not showing up for exams for an unknown reason”.
After leaving the seminary, Stalin worked in a weather office until, in 1901, when he devoted himself to boot-strapping underground revolutionary.
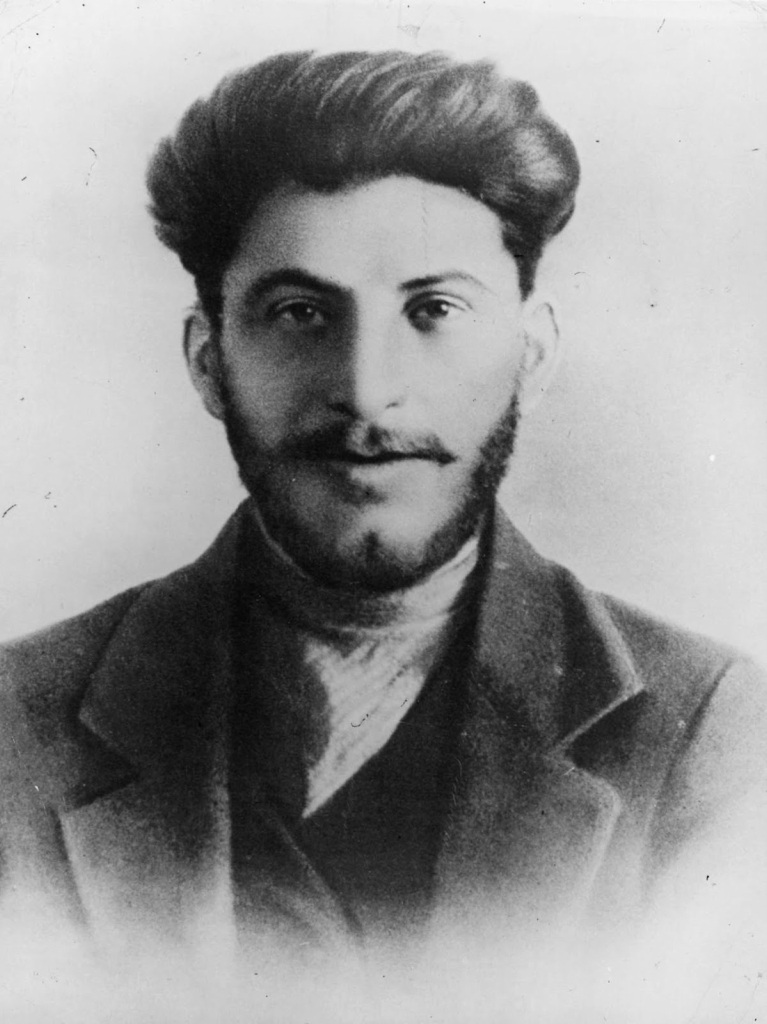
The secret police of the Russian Empire caught Joseph Stalin three times upon his tries to escape the exile.
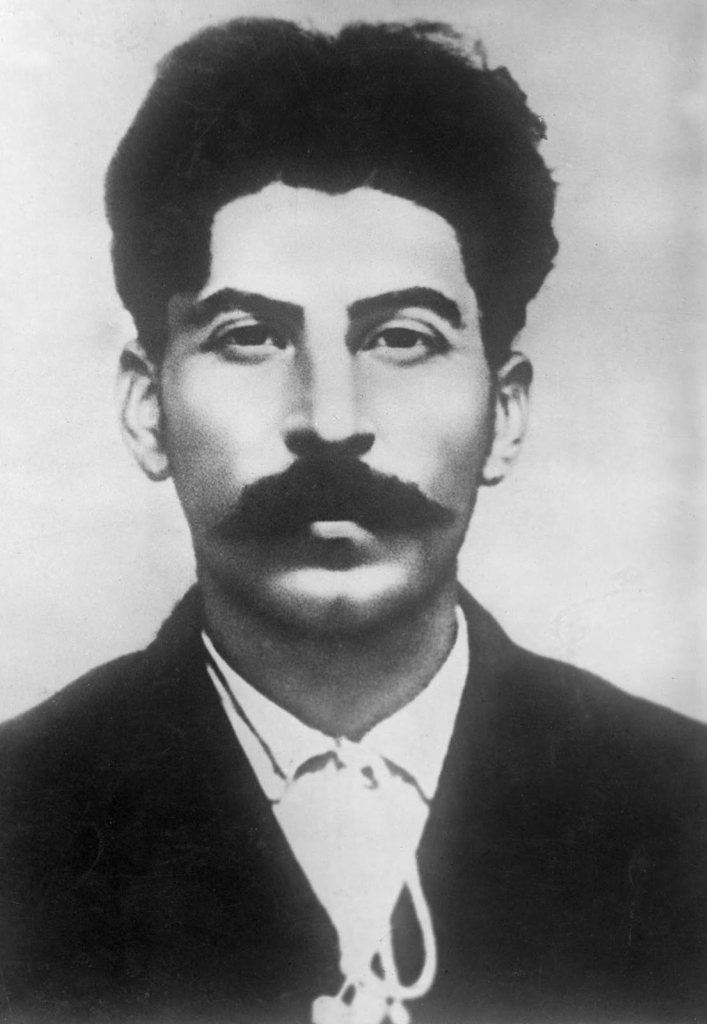
From the police archives, 1909
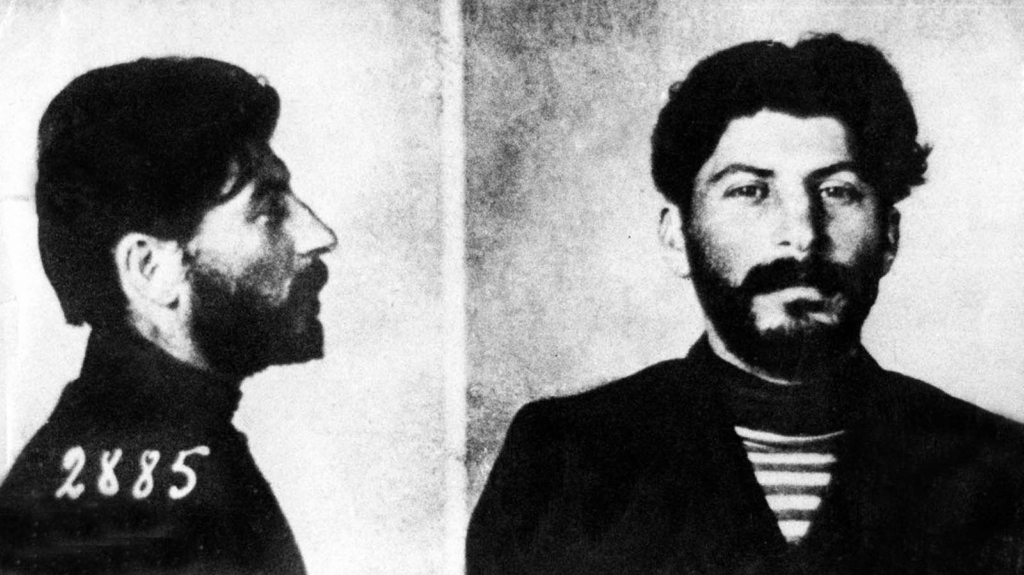
From the police archives, 1910
A bright revolutionary start
The name of Stalin became widespread in September 1901, when a young illegal newspaper, “Brdzola” (“Struggle”) published his article on the front page. Later the authority of the Russian Social-Democratic party involved 22-years old revolutionary into the organization of their new Batumi branch.
This party split in 1903 into more and less radical branches. Young Stalin joined the more radical group of Bolsheviks. Overal he triggered more aggressive revolutionary activities within the Bolsheviks wing of the party. He contributed to the party fundraising actively. Stalin organized several robberies and blackmailed — even kidnapping.
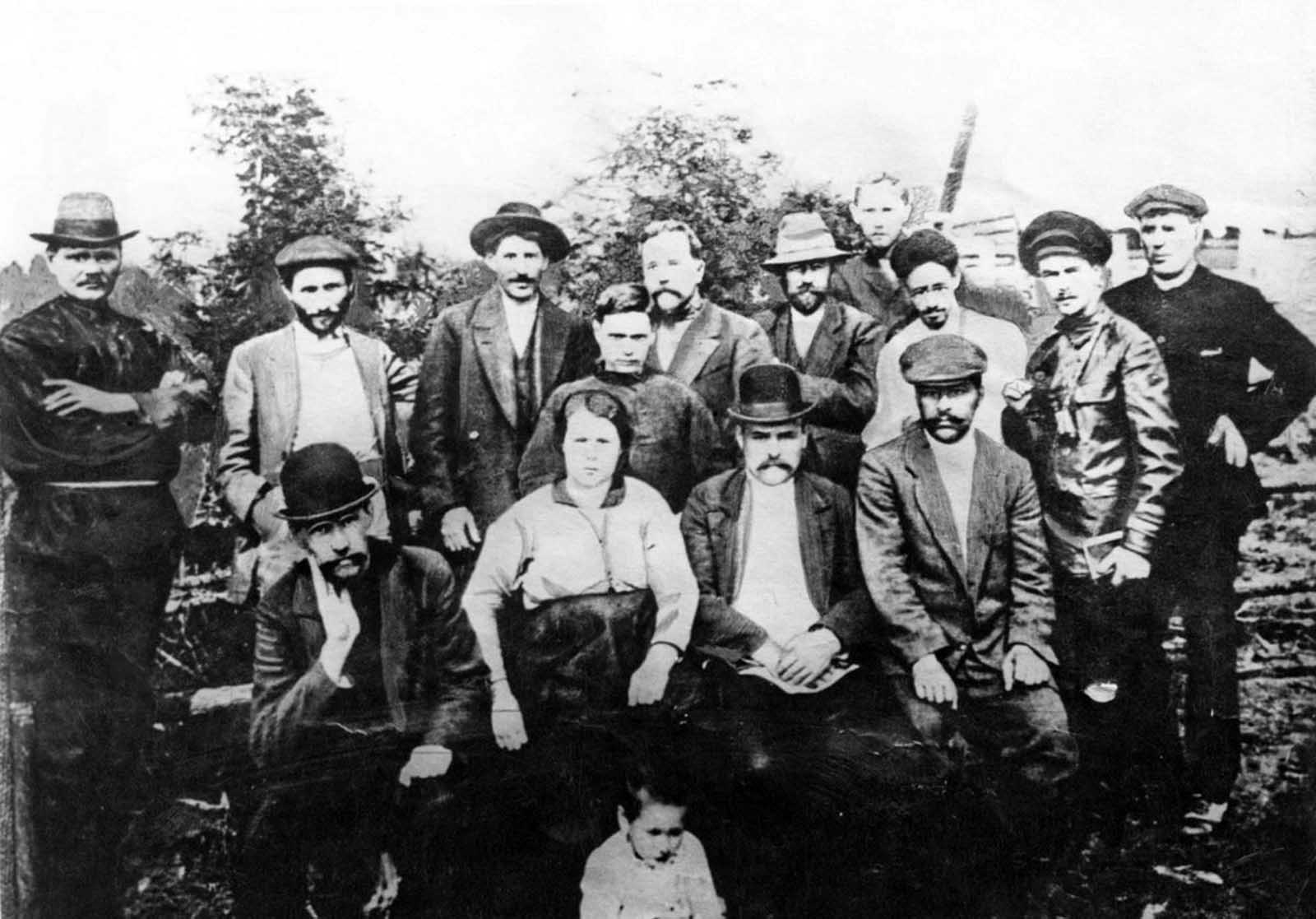
Stalin (standing, third from left) with a group of Bolshevik revolutionaries in Turukhansk, Russian Empire. 1915.
In 1904, Joseph organized a grand strike of workers in the oil fields in Baku. This protest ended up with the agreement between the strikers and owners. It was a massive success for a young revolutionary. Communists’ party career of young Stalin skyrocketed. Joseph attended the 1st conference of the Bolshevik’s party in Tammerfors (Finland), where he first personally met Lenin. Stalin traveled to Stockholm next year while attending the Communist’s event. It was his first trip abroad.
Young Stalin didn’t forget about his personal life while building a communist career. On the night of July 16, 1906, Joseph Dzhugashvili got married to Ekaterina Svanidze. Stalin’s first son, Yakov, was born in 1907. Stalin’s wife died of typhus at the end of the same year.
Meanwhile, Stalin continued to prove his reputation as a productive communist party member. He visited several Bolshevik’s events, including one in London. He appeared on the radars of the Russian police as a result. Stalin was twice in exile in the town of Solvychegodsk, Vologda province – from February 27 to June 24, 1909, and from October 29, 1910, to July 6, 1911. Joseph escaped the exile twice, but police officers arrested him again all the time.
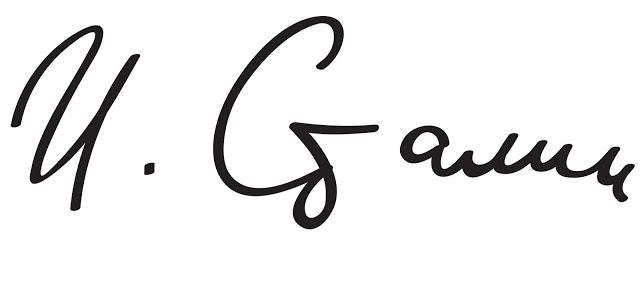
Signature of Joseph Stalin from his forged passport. This fake document allowed Joseph to escape from his 3rd exile in Syberia.
The Syberia home of the name Stalin
Joseph used both Stalin and Djugashvili names until 1912. He renamed himself ultimately during the exile in Siberia. This time, the place of exile was the city of Narym, Tomsk province. It was a harsh and cold place in the heart of Syberia. Stalin spent 41 days in Narym – from July 22 to September 1, 1912. He managed to bribe the secret police and escape the exile. Joseph used a forged passport with a Stalin name to board a train and directed to the European part of Russia. He became a founding member of the Pravda newspaper during this period.
Police arrested Stalin the fourth time in March 1913. The secret police were very cautious this time and managed to keep him in exile until 1916.
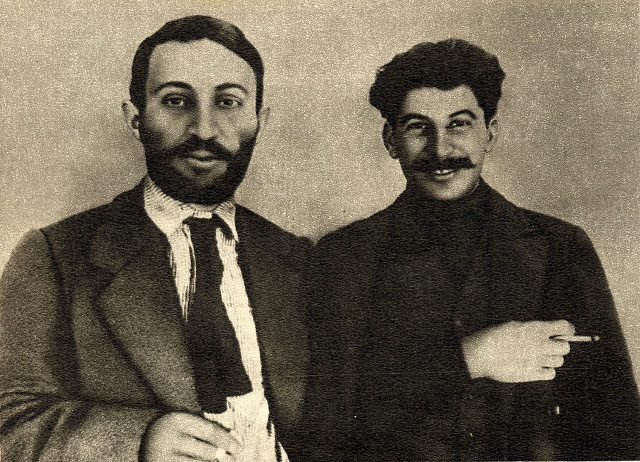
Joseph Stalin and Suren Spandarjan, 1915
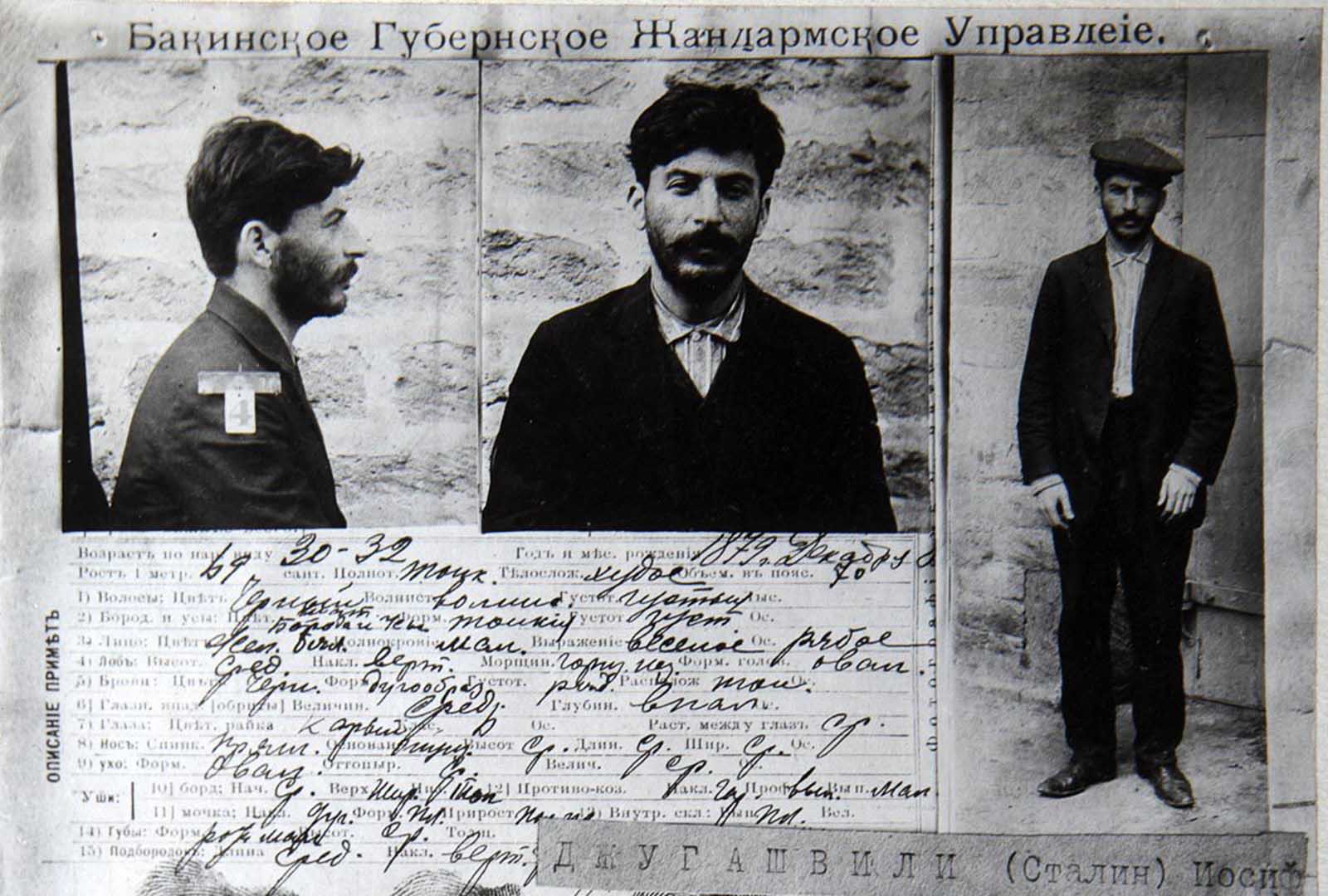
A criminal file on Stalin following his arrest in Baku, Azerbaijan. 1910.
A fresh start
February Revolution set Stalin free. He hurried back to St. Petersburg to meet Lenin again from Switzerland. Meanwhile, Joseph became a member of the editorial board of the newspaper Pravda.
Stalin supported Lenin’s concept of transforming the “bourgeois-democratic” February revolution into a proletarian socialist revolution. Stalin joined the Central Committee of the Bolshevik’s party in April. He participated in the First All-Russian Congress of Soviet Workers and Soldiers; was elected a member of the All-Russian Central Executive Committee and a member of the Bureau of the All-Russian Central Executive Committee from the Bolshevik faction.
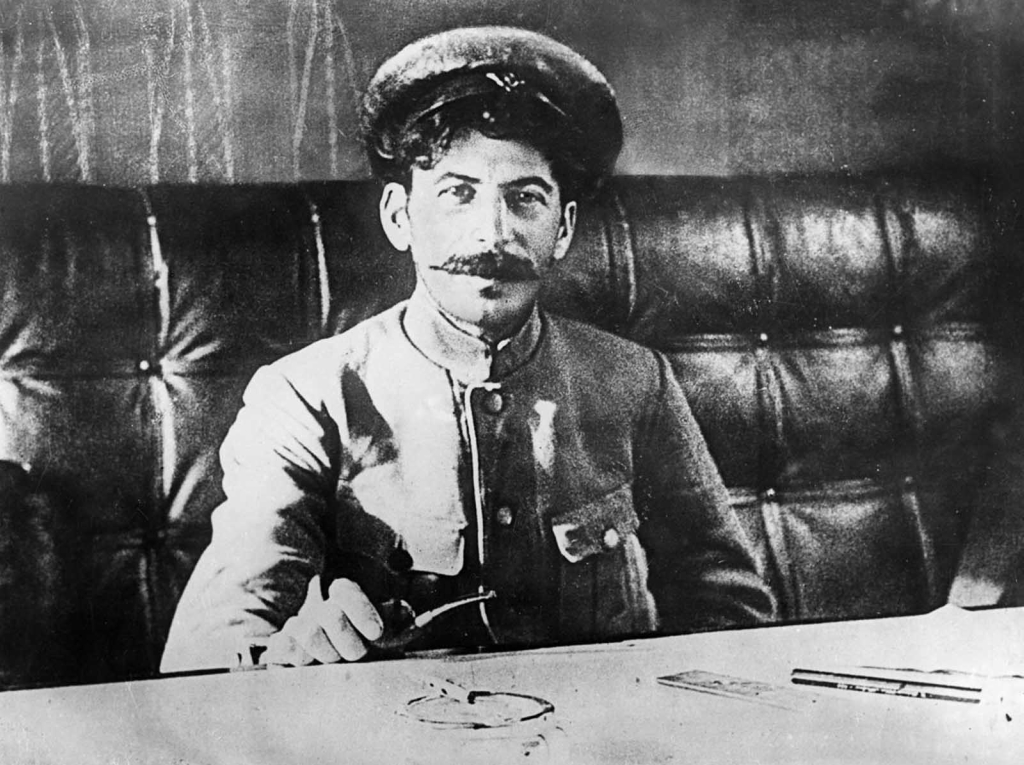
Stalin during the October Revolution in Russia, 1917
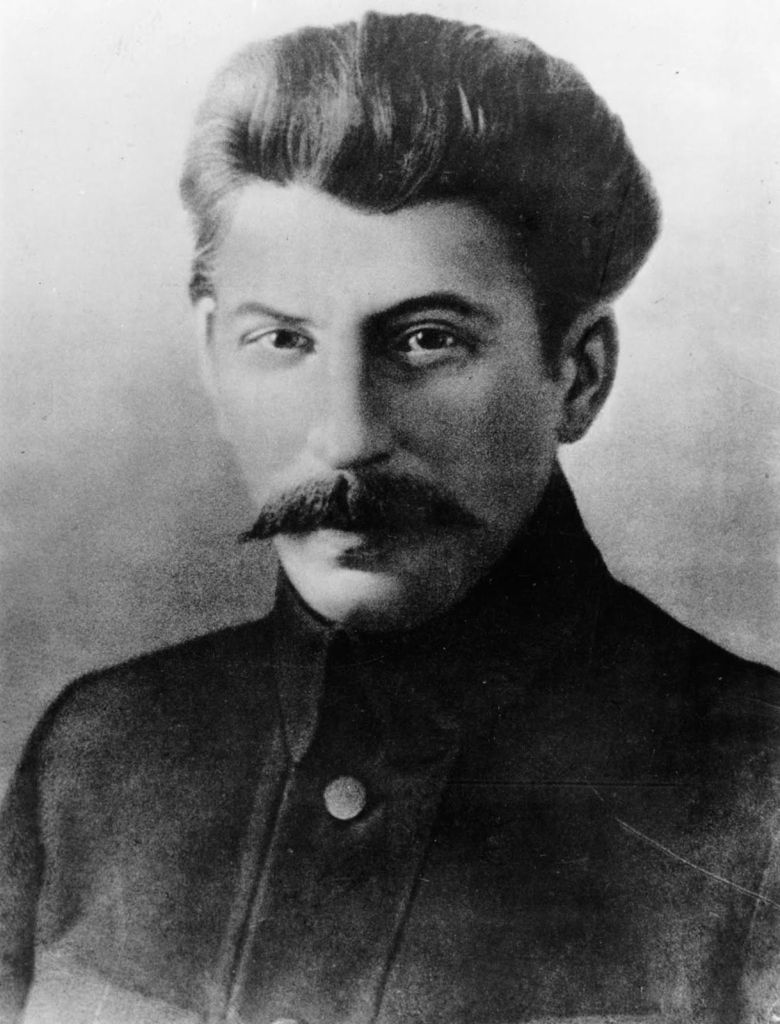
Stalin, in 1918, still looks pretty fresh.
At this time, Stalin and Trotsky held a conference of the Bolsheviks – delegates to the 2nd All-Russian Congress of Soviets of the RSD. Stalin delivered a report on ongoing political events. He participated in a meeting of the Central Committee of the Communistic party that formed the structure and name of the new Soviet government.
After the victory of the October Revolution, Stalin entered the Council of People’s Commissars (SNK) as People’s Commissar for Nationalities (Stalin wrote the article “Marxism and the National Policies” at the end of 1912-1913, and from that time he gained the reputation of national problems expert).
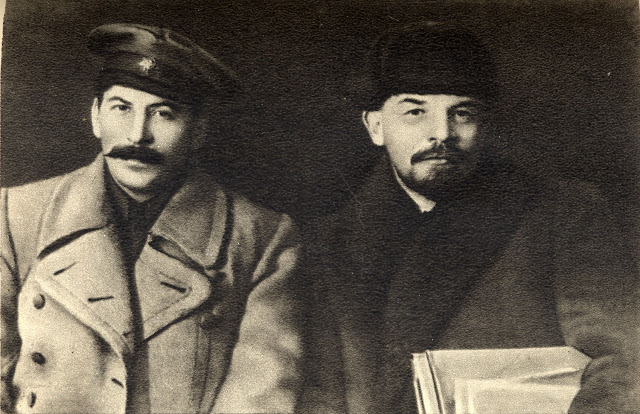
Joseph Stalin and Vladimir Lenin during the VIII Congress of the Party, 1919
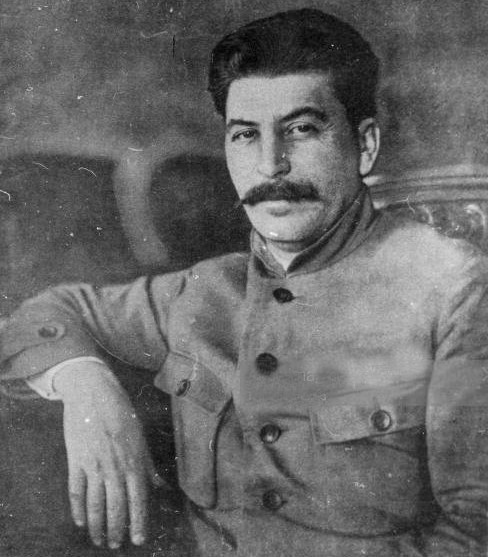
Joseph Stalin in 1920
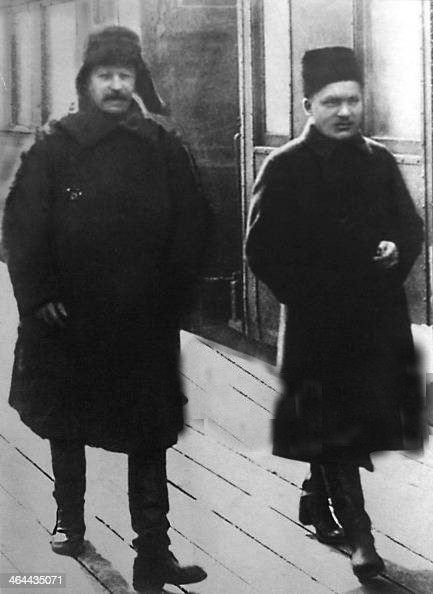
Stalin and Kalinin, 1919
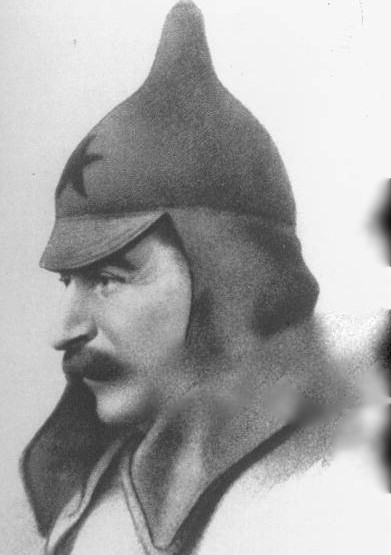
During the Russian Revolution, 1918
Stalin’s way to power
Joesph Stalin entered the Bureau of the Central Committee of the Communist Party, together with Lenin, Trotsky, and Sverdlov. This body had “the right to decide all urgent matters, but with the obligatory involvement of all members of the Central Committee who were at that moment in the central party office.”
Stalin married again in 1918, and his son Vasily was born on March 24, 1921, in Moscow. In 1922 Stalin was elected as a general secretary of the Communist Party. Initially, this position meant only the leadership of the party structure. Lenin was the Chairman of the Council of People’s Commissars and led the government. But in fact, the position of the general secretary had a crucial role in the developing structure of the Communist party. Stalin solely appointed numerous mid-level bureaucrats, which in their turn elected the government. Here’s how Stalin accumulated the real power in post-revolution Russia.
[…] from the early years of young Mao Zedong lived to our days. But Oldpics has already published old photographs of Joseph Stalin, Winston Churchill, and even some pictures of Adolf Hitler. So we had to find at least some images […]
[…] early photographs of the great politicians of the 20th century. You can check noteworthy photos of young Joseph Stalin, rare images of Mao Zedong, and even pictures of Adolf Hitler during his WWI […]